Khi lập trình web với ASP.NET MVC hay bất kỳ ngôn ngữ nào khác như PHP chẳng hạn, chúng ta đều gặp những tình huống liên quan đến Upload file lên hosting, dễ thấy nhất là upload hình sản phẩm cho một sản phẩm nào đó.
Với ASP.NET MVC chúng ta dễ dàng làm được điều đó, bạn có thể upload 1 file hoặc upload nhiều file (Multiple file upload) trong ASP.NET MVC cùng lúc. Nó cực kỳ hữu ích trong nhiều trường hợp. Đoạn code nhỏ sau đây sẽ minh họa cho vấn đề này.
- Bạn có thể xem thêm: Upload file trong ASP.NET
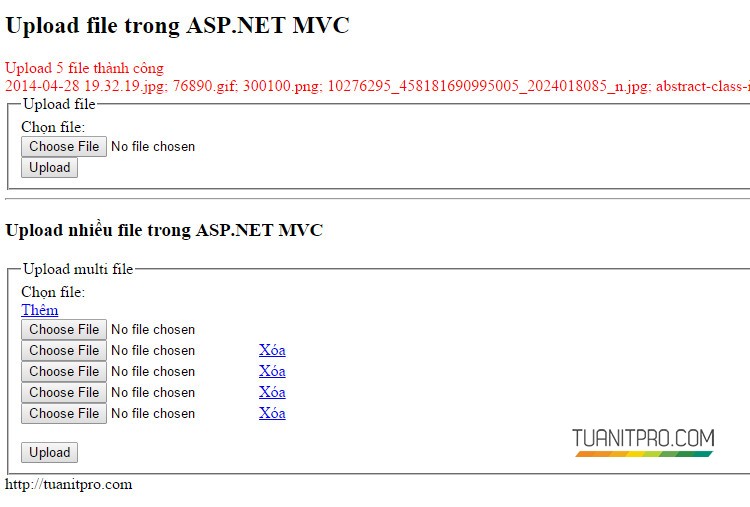
Code HTML
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width" /> <title>Upload file trong ASP.NET MVC</title> <script src="//code.jquery.com/jquery-1.11.0.min.js"></script> <script type="text/javascript"> $(document).ready(function () { var max_fields = 10; //maximum input boxes allowed var wrapper = $(".myinput"); //Fields wrapper var add_button = $(".btnAddNew"); //Add button ID var x = 0; //initlal text box count $(add_button).click(function (e) { //on add input button click e.preventDefault(); if (x < max_fields) { //max input box allowed x++; //text box increment $(wrapper).append('<div> <input type="file" name="uploadFile['+x+']" /><a href="#" class="btnRemove">Xóa</a></div>'); //add input box } }); $(wrapper).on("click", ".btnRemove", function (e) { //user click on remove text e.preventDefault(); $(this).parent('div').remove(); x--; }) }); </script> </head> <body> <div> <h2>Upload file trong ASP.NET MVC</h2> <div style="color:red"> @Html.Raw(TempData["Msg"]) </div> <fieldset> <legend>Upload file</legend> @using (Html.BeginForm("Upload", "Upload", FormMethod.Post, new { enctype = "multipart/form-data" })) { <label>Chọn file: </label> <br /> <input type="file" name="uploadFile" required /><br /> <input type="submit" value="Upload" /> } </fieldset> <hr /> <h3>Upload nhiều file trong ASP.NET MVC</h3> <fieldset> <legend>Upload multi file</legend> @using (Html.BeginForm("UploadMulti", "Upload", FormMethod.Post, new { enctype = "multipart/form-data" })) { <label>Chọn file: </label><br /> <a class="btnAddNew" href="#">Thêm</a> <br /> <div id="myinput" class="myinput"> <input type="file" name="uploadFile[0]" required /><br /> </div> <br /> <input type="submit" value="Upload" /> } </fieldset>
Code cs
/** FileName: UploadController.cs Project Name: DateTime Ajax Date Created: 12/17/2014 11:30:58 PM Description: File Upload trong ASP.NET MVC Version: 0.0.0.0 Author: Lê Thanh Tuấn - Khoa CNTT Author Email: [email protected] Author Mobile: 0976060432 Author URI: https://tuanitpro.com License: */ public class UploadController : Controller { // GET: Upload public ActionResult Index() { return View(); } [HttpPost] public ActionResult Upload(HttpPostedFileBase uploadFile) { if (ModelState.IsValid) { string filePath = Path.Combine(HttpContext.Server.MapPath("/Uploads/demo"), Path.GetFileName(uploadFile.FileName)); uploadFile.SaveAs(filePath); TempData["Msg"] = string.Format("Upload file {0} thành công", uploadFile.FileName); } return RedirectToAction("Index"); } [HttpPost] public ActionResult UploadMulti(List<HttpPostedFileBase> uploadFile) { string abc = ""; string def = ""; foreach (var item in uploadFile) { string filePath = Path.Combine(HttpContext.Server.MapPath("/Uploads/demo"), Path.GetFileName(item.FileName)); item.SaveAs(filePath); abc = string.Format("Upload {0} file thành công", uploadFile.Count); def += item.FileName + "; "; } TempData["Msg"] = abc + "</br>" + def; return RedirectToAction("Index"); } }
Live demo
Chúc các bạn thành công.