Chào các bạn
Khi lập trình các bạn hay gặp vấn đề khó khăn khi lấy dữ liệu giữa các Dropdown List khác nhau. Ví dụ: Từ tỉnh thành -> quận huyện -> Xã phường…
Đây là khó khăn của rất nhiều bạn sinh viên khi mới bắt đầu học lập trình. Video dưới đây sẽ hướng dẫn bạn cách giải quyết, khá là đơn giản. Hoàn toàn có thể áp dụng nhiều trường hợp khác nhau.
Video không có tiếng, do mình làm trong lúc ngẫu hứng ngoài quán cafe. Các bạn có thể theo dõi, hoặc tải mã nguồn về phân tích thêm.
Slide giới thiệu: https://docs.google.com/presentation/d/1soxUWN7Xa6Z89Az-PNCtiyGgMVdVSgmqzp1bf7hdi50/edit?usp=sharing
Tài nguyên
SQL Data:
CSDL tên Quốc gia, tỉnh thành phố thị xã quận huyện, xã phường Việt Nam
Demo
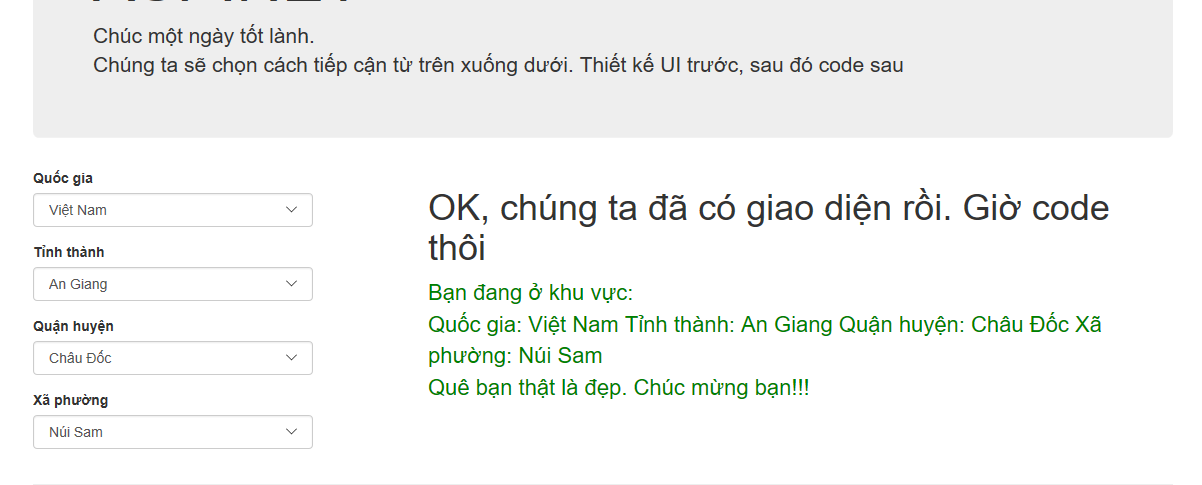
Code C#
public class MyDbContext:DbContext { // Ở đây các bạn có thể khai báo trong Web.config. private const string sqlConnection = @"Data Source=.\SQLExpress; Initial Catalog = CountryDb; User Id=sa; Password = sa"; public MyDbContext() : base(sqlConnection) { } public DbSet<Country> Countries { get; set; } public DbSet<Province> Provinces { get; set; } public DbSet<District> Districts { get; set; } public DbSet<Ward> Wards { get; set; } }
public JsonResult GetAllCountries() { using(var db = new MyDbContext()) { var data = db.Countries.OrderBy(x=>x.Name).ToList(); return Json(data, JsonRequestBehavior.AllowGet); } } /// <summary> /// Hàm lấy danh sách tỉnh thành theo CountryId. /// Id = 237 là của Việt Nam. Do database mình quy định vậy /// Test OK /// </summary> /// <param name="id">Id của country</param> /// <returns></returns> public JsonResult GetAllProvinceByCountryId(int? id=237) { using (var db = new MyDbContext()) { var data = db.Provinces.Where(x=>x.CountryId== id).OrderBy(x=>x.Name).ToList(); return Json(data, JsonRequestBehavior.AllowGet); } } /// <summary> /// Hàm lấy tất cả danh sách quận huyện /// Id = 1 là Hà Nội, do database mình quy định vậy /// Test OK /// </summary> /// <param name="id">Id = ProvinceId</param> /// <returns></returns> public JsonResult GetAllDistrictByProvinceId(int? id = 1) { using (var db = new MyDbContext()) { var data = db.Districts.Where(x => x.ProvinceId == id).OrderBy(x=>x.Name).ToList(); return Json(data, JsonRequestBehavior.AllowGet); } } /// <summary> /// Hàm lấy danh sách xã phường theo quận huyện /// Id= 1 là Ba Đình. Do database quy định /// Test OK /// </summary> /// <param name="id"></param> /// <returns></returns> public JsonResult GetAllWardByDistrictId(int? id = 1) { using (var db = new MyDbContext()) { var data = db.Wards.Where(x => x.DistrictId == id).OrderBy(x=>x.Name).ToList(); return Json(data, JsonRequestBehavior.AllowGet); } }
Code js
// File javascript để lấy dữ liệu // Khai báo URL service của bạn ở đây var baseService = "/Service"; var countryUrl = baseService + "/GetAllCountries"; var provinceUrl = baseService + "/GetAllProvinceByCountryId"; var districtUrl = baseService + "/GetAllDistrictByProvinceId"; var wardUrl = baseService + "/GetAllWardByDistrictId"; $(document).ready(function () { // load danh sách country _getCountries(); $("#ddlCountry").on('change', function () { var id = $(this).val(); if (id != undefined && id != '') { _getProvince(id); } }); $("#ddlProvince").on('change', function () { var id = $(this).val(); if (id != undefined && id != '') { _getDistrict(id); } }); $("#ddlDistrict").on('change', function () { var id = $(this).val(); if (id != undefined && id != '') { _getWard(id); } }); $("#ddlWard").on('change', function () { var countryText = $("#ddlCountry option:selected").text(); var provinceText = $("#ddlProvince option:selected").text(); var districtText = $("#ddlDistrict option:selected").text(); var wardText = $("#ddlWard option:selected").text(); var html = "Quốc gia: " + countryText + " Tỉnh thành: " + provinceText + " " + "Quận huyện: " + districtText + " " + "Xã phường: " + wardText; html += "</br>Quê bạn thật là đẹp. Chúc mừng bạn!!!"; $("#divResult").html(html); }); }); function _getCountries() { $.get(countryUrl, function (data) { if (data != null && data != undefined && data.length) { var html = ''; html += '<option value="">--Không chọn--</option>'; $.each(data, function (key, item) { html += '<option value=' + item.Id + '>' + item.Name + '</option>'; }); $("#ddlCountry").html(html); } }); } // truyền id của country vào function _getProvince(id) { $.get(provinceUrl + "/"+id, function (data) { if (data != null && data != undefined && data.length) { var html = ''; html += '<option value="">--Không chọn--</option>'; $.each(data, function (key, item) { html += '<option value=' + item.Id + '>' + item.Name + '</option>'; }); $("#ddlProvince").html(html); } }); } // truyền id của province vào function _getDistrict(id) { $.get(districtUrl + "/" + id, function (data) { if (data != null && data != undefined && data.length) { var html = ''; html += '<option value="">--Không chọn--</option>'; $.each(data, function (key, item) { html += '<option value=' + item.Id + '>' + item.Name + '</option>'; }); $("#ddlDistrict").html(html); } }); } // truyền id của district vào function _getWard(id) { $.get(wardUrl + "/" + id, function (data) { if (data != null && data != undefined && data.length) { var html = ''; html += '<option value="">--Không chọn--</option>'; $.each(data, function (key, item) { html += '<option value=' + item.Id + '>' + item.Name + '</option>'; }); $("#ddlWard").html(html); } }); }
Chúc các bạn thành công.
Download GitHub